在现代软件开发中,安全性始终是一个至关重要的考虑因素。本文将介绍一些编写安全的Go代码的最佳实践,以帮助开发人员构建更加安全、可靠的应用程序。
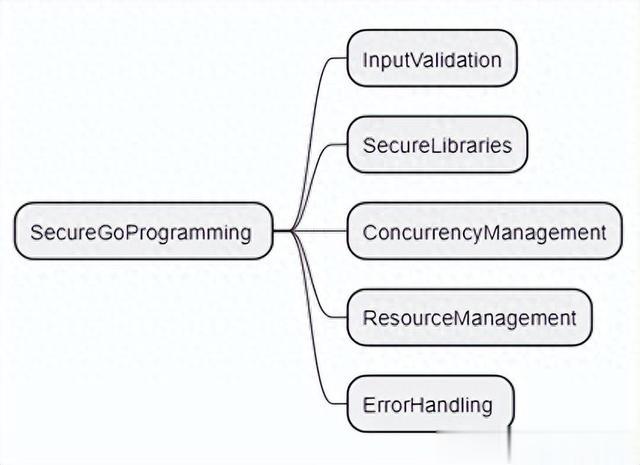
输入验证是编写安全代码的第一步。确保所有用户输入的数据都经过严格的验证和清理,以防止常见的攻击如SQL注入、XSS(跨站脚本攻击)等。
package mainimport ( "net/http" "regexp")func validateInput(input string) bool { // 仅允许字母和数字 var validInput = regexp.MustCompile(`^[a-zA-Z0-9]+$`) return validInput.MatchString(input)}func handler(w http.ResponseWriter, r *http.Request) { userInput := r.URL.Query().Get("input") if !validateInput(userInput) { http.Error(w, "Invalid input", http.StatusBadRequest) return } // 处理有效输入}func main() { http.HandleFunc("/", handler) http.ListenAndServe(":8080", nil)}2. 使用安全的标准库Go的标准库提供了许多安全功能,如加密、哈希、验证等。使用这些库可以减少编写和维护安全代码的复杂性。
package mainimport ( "crypto/aes" "crypto/cipher" "crypto/rand" "io" "fmt")func encrypt(data []byte, passphrase string) ([]byte, error) { block, err := aes.NewCipher([]byte(passphrase)) if err != nil { return nil, err } ciphertext := make([]byte, aes.BlockSize+len(data)) iv := ciphertext[:aes.BlockSize] if _, err := io.ReadFull(rand.Reader, iv); err != nil { return nil, err } stream := cipher.NewCFBEncrypter(block, iv) stream.XORKeyStream(ciphertext[aes.BlockSize:], data) return ciphertext, nil}func main() { plaintext := []byte("This is a secret message") passphrase := "mysecretpassword" encrypted, err := encrypt(plaintext, passphrase) if err != nil { fmt.Println("Error encrypting:", err) return } fmt.Printf("Encrypted message: %x\n", encrypted)}3. 防止并发问题Go以其并发能力著称,但如果不小心使用,可能会引入并发问题。使用互斥锁(mutex)和通道(channel)来管理并发操作,避免竞态条件。
package mainimport ( "fmt" "sync")var counter intvar mutex = &sync.Mutex{}func increment() { mutex.Lock() defer mutex.Unlock() counter++}func main() { var wg sync.WaitGroup for i := 0; i < 1000; i++ { wg.Add(1) go func() { defer wg.Done() increment() }() } wg.Wait() fmt.Println("Final counter value:", counter)}4. 使用defer确保资源释放Go的defer语句可以确保资源在函数结束时被正确释放,从而避免资源泄漏问题。
package mainimport ( "database/sql" _ "github.com/go-sql-driver/mysql" "log")func main() { db, err := sql.Open("mysql", "user:password@/dbname") if err != nil { log.Fatal(err) } defer db.Close() err = db.Ping() if err != nil { log.Fatal(err) } // 使用数据库...}5. 检查错误Go语言的错误处理机制要求开发者显式检查和处理每一个错误。通过良好的错误处理,可以避免程序在意外情况下崩溃。
package mainimport ( "fmt" "os")func main() { file, err := os.Open("nonexistent.txt") if err != nil { fmt.Println("Error opening file:", err) return } defer file.Close() // 处理文件内容...}6. 使用context控制超时和取消在网络请求或其他长时间运行的操作中,使用context包来管理超时和取消操作,以提高程序的健壮性。
package mainimport ( "context" "fmt" "time")func main() { ctx, cancel := context.WithTimeout(context.Background(), 2*time.Second) defer cancel() select { case <-time.After(3 * time.Second): fmt.Println("Operation completed") case <-ctx.Done(): fmt.Println("Operation timed out") }}结论编写安全的Go代码需要开发人员在多个方面进行细致的考虑和实践。通过输入验证、使用安全的标准库、防止并发问题、确保资源释放、检查错误和使用context管理操作,可以大幅提升Go应用程序的安全性和可靠性