今天分享一些Python 中短小精悍的语法技巧,能让你写出更简洁优雅的代码:
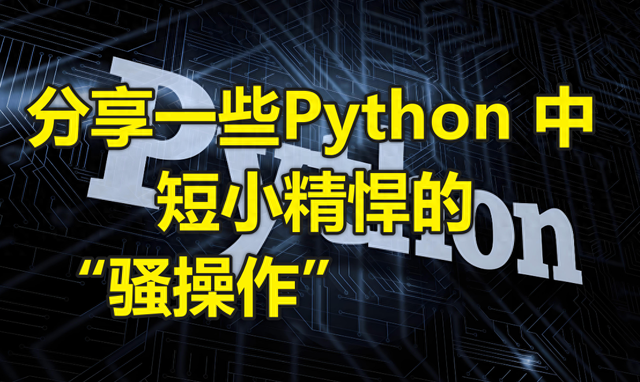
good good study, day day up!
1.列表推导式 + 条件过滤# 生成偶数的平方squares = [x**2 for x in range(10) if x % 2 == 0]# 结果:[0, 4, 16, 36, 64]2.三元表达式# 单行条件赋值result = "Yes" if 5 > 3 else "No"# 结果:"Yes"3.交换变量值a, b = 5, 10a, b = b, a # 无需临时变量print(a, b) # 10 54.合并字典 (Python 3.9+)dict1 = {"a": 1, "b": 2}dict2 = {"b": 3, "c": 4}merged = dict1 | dict2 # {'a':1, 'b':3, 'c':4}5.快速反转字符串/列表s = "hello"print(s[::-1]) # "olleh"lst = [1, 2, 3]print(lst[::-1]) # [3, 2, 1]6.同时获取索引和值 (enumerate)for index, value in enumerate(["a", "b", "c"]): print(index, value)# 0 a# 1 b# 2 c7.合并两个列表成字典 (zip)keys = ["a", "b", "c"]values = [1, 2, 3]d = dict(zip(keys, values)) # {'a':1, 'b':2, 'c':3}8.海象运算符 (Python 3.8+)# 在表达式中赋值if (n := len("hello")) > 3: print(f"长度是{n}") # 长度是59.展开多层嵌套列表nested = [[1, 2], [3, 4], [5]]flat = [num for sublist in nested for num in sublist]# [1, 2, 3, 4, 5]10.快速去重并保持顺序lst = [3, 1, 2, 3, 2]unique = list(dict.fromkeys(lst)) # [3, 1, 2]11.链式比较x = 5print(3 < x < 10) # True (等价于 3 < x and x < 10)12.f-string 调试 (Python 3.8+)value = 42print(f"{value=}") # 输出 value=4213.多变量赋值a, *b, c = [1, 2, 3, 4, 5]print(a, b, c) # 1 [2,3,4] 514.生成器表达式省内存# 不会立即生成所有元素sum_squares = sum(x**2 for x in range(1000000))15.用_忽略不需要的值# 解包时忽略某些值a, _, c = (1, 2, 3) # a=1, c=316.字典的setdefaultd = {}for k, v in [("a", 1), ("a", 2)]: d.setdefault(k, []).append(v)# {'a': [1, 2]}17.上下文管理器打开文件with open("file.txt") as f: content = f.read()# 自动关闭文件18.any/all快速判断nums = [2, 4, 6, 8]print(all(n%2 ==0 for n in nums)) # Trueprint(any(n >10 for n in nums)) # False19.用*解包参数def sum(a, b, c): return a + b + cvalues = [1, 2, 3]print(sum(*values)) # 620.itertools模块黑魔法from itertools import permutations, combinations# 排列组合print(list(permutations("ABC", 2))) # [('A','B'), ('A','C'), ...]print(list(combinations("ABC", 2))) # [('A','B'), ('A','C'), ...]注意:可读性优先,不要为了炫技而过度使用!