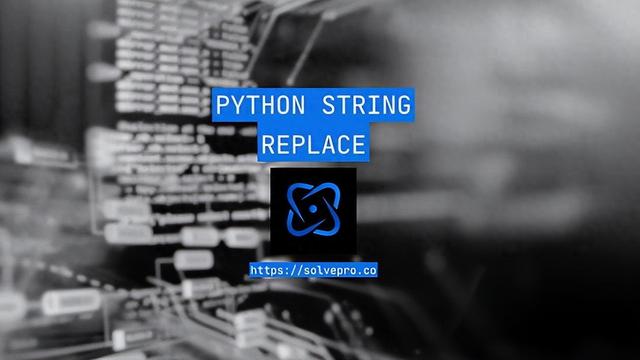
作为开发人员,几乎每天都会使用 Python 中的字符串替换。无论您是清理数据、设置文本格式还是构建搜索功能,了解如何有效地替换文本都将使您的代码更简洁、更高效。
String Replace 的基础知识'replace()' 方法适用于任何字符串,语法简单:
text = "Hello world"new_text = text.replace("world", "Python")print(new_text) # Output: Hello Python您还可以指定要进行的替换数量:
text = "one two one two one"# Replace only the first two occurrencesresult = text.replace("one", "1", 2)print(result) # Output: 1 two 1 two one实际应用清理数据以下是从 CSV 文件中清理杂乱数据的方法:
def clean_data(text): # Remove extra whitespace text = text.replace("\t", " ") # Standardize line endings text = text.replace("\r\n", "\n") # Fix common typos text = text.replace("potatoe", "potato") # Standardize phone number format text = text.replace("(", "").replace(")", "").replace("-", "") return textdata = """Name\tPhoneJohn Doe\t(555)-123-4567Jane Smith\t(555)-987-6543"""clean = clean_data(data)print(clean)URL 处理使用 URL 时,您通常需要替换特殊字符:
def format_url(url): # Replace spaces with URL-safe characters url = url.replace(" ", "%20") # Replace backslashes with forward slashes url = url.replace("\\", "/") # Ensure protocol is consistent url = url.replace("http://", "https://") return urlmessy_url = "http://example.com/my folder\\documents"clean_url = format_url(messy_url)print(clean_url) # Output: https://example.com/my%20folder/documents文本模板系统创建一个简单的模板系统来个性化消息:
def fill_template(template, **kwargs): result = template for key, value in kwargs.items(): placeholder = f"{{{key}}}" result = result.replace(placeholder, str(value)) return resulttemplate = "Dear {name}, your order #{order_id} will arrive on {date}."message = fill_template( template, name="Alice", order_id="12345", date="Monday")print(message) # Output: Dear Alice, your order #12345 will arrive on Monday.高级替换技术链式替换有时您需要按顺序进行多次替换:
def normalize_text(text): replacements = { "ain't": "is not", "y'all": "you all", "gonna": "going to", "wanna": "want to" } result = text for old, new in replacements.items(): result = result.replace(old, new) return resulttext = "Y'all ain't gonna believe what I wanna show you!"print(normalize_text(text))# Output: You all is not going to believe what want to show you!区分大小写的替换当大小写很重要时,您可能需要不同的方法:
def smart_replace(text, old, new, case_sensitive=True): if case_sensitive: return text.replace(old, new) # Case-insensitive replacement index = text.lower().find(old.lower()) while index != -1: text = text[:index] + new + text[index + len(old):] index = text.lower().find(old.lower(), index + len(new)) return text# Example usagetext = "Python is great. PYTHON is amazing. python is fun."result = smart_replace(text, "python", "Ruby", case_sensitive=False)print(result) # Output: Ruby is great. Ruby is amazing. Ruby is fun.使用特殊字符处理特殊字符时,请小心转义序列:
def clean_file_path(path): # Replace Windows-style paths with Unix-style path = path.replace("\\", "/") # Remove illegal characters illegal_chars = '<>:"|?*' for char in illegal_chars: path = path.replace(char, "_") # Replace multiple slashes with single slash while "//" in path: path = path.replace("//", "/") return pathpath = "C:\\Users\\JohnDoe\\My:Files//project?docs"clean_path = clean_file_path(path)print(clean_path) # Output: C/Users/JohnDoe/My_Files/project_docs性能提示批量替换进行多次替换时,一次执行所有替换会更快:
import redef batch_replace(text, replacements): # Create a regular expression pattern for all keys pattern = '|'.join(map(re.escape, replacements.keys())) # Replace all matches using a single regex return re.sub(pattern, lambda m: replacements[m.group()], text)text = "The quick brown fox jumps over the lazy dog"replacements = { "quick": "slow", "brown": "black", "lazy": "energetic"}result = batch_replace(text, replacements)print(result) # Output: The slow black fox jumps over the energetic dog节省内存的替换对于大文件,请逐行处理它们:
def process_large_file(input_file, output_file, old, new): with open(input_file, 'r') as fin, open(output_file, 'w') as fout: for line in fin: fout.write(line.replace(old, new))# Example usageprocess_large_file('input.txt', 'output.txt', 'old_text', 'new_text')常见问题和解决方案替换行尾请小心不同作系统中的行尾:
def normalize_line_endings(text): # First, standardize to \n text = text.replace('\r\n', '\n') text = text.replace('\r', '\n') # Remove empty lines while '\n\n\n' in text: text = text.replace('\n\n\n', '\n\n') return texttext = "Line 1\r\nLine 2\rLine 3\n\n\nLine 4"normalized = normalize_line_endings(text)print(normalized)处理 Unicode使用 Unicode 文本时,请注意字符编码:
def clean_unicode_text(text): # Replace common Unicode quotation marks with ASCII ones replacements = { '"': '"', # U+201C LEFT DOUBLE QUOTATION MARK '"': '"', # U+201D RIGHT DOUBLE QUOTATION MARK ''': "'", # U+2018 LEFT SINGLE QUOTATION MARK ''': "'", # U+2019 RIGHT SINGLE QUOTATION MARK } for old, new in replacements.items(): text = text.replace(old, new) return textfancy_text = "Here's some "fancy" text"plain_text = clean_unicode_text(fancy_text)print(plain_text) # Output: Here's some "fancy" text请记住,Python 中的字符串替换作会创建新字符串,它们不会修改原始字符串。这在处理大型文本或 in Loop 时非常重要。如果需要进行多次替换,请考虑使用正则表达式或批处理以获得更好的性能。
此外,虽然 'replace()' 非常适合简单的字符串替换,但对于更复杂的模式匹配和替换,请查看 Python 的 're' 模块,该模块通过正则表达式提供更高级的文本处理功能。