在许多应用程序中,特别是在需要快速访问或激活特定功能的情况下,全局热键(即在操作系统的任何地方都可以使用的快捷键)变得非常有用。在C#中实现这一功能可能看起来有些复杂,但通过正确的步骤和Windows API的帮助,这实际上是完全可行的。本文将指导你如何在C#应用程序中创建和使用全局热键。
步骤1:定义API函数首先,我们需要定义一些Windows API函数,这些函数将帮助我们注册和注销全局热键。通过使用DllImport属性,我们可以在C#中调用这些本地方法。
using System;using System.Runtime.InteropServices;using System.Windows.Forms;public HotKeyManager{ // 导入user32.dll中的RegisterHotKey函数,用于注册全局热键 [DllImport("user32.dll", SetLastError = true)] private static extern bool RegisterHotKey(IntPtr hWnd, int id, uint fsModifiers, uint vk); // 导入user32.dll中的UnregisterHotKey函数,用于注销全局热键 [DllImport("user32.dll", SetLastError = true)] private static extern bool UnregisterHotKey(IntPtr hWnd, int id); // 定义WM_HOTKEY消息的消息ID,当注册的热键被按下时,系统发送此消息 private const int WM_HOTKEY = 0x0312; // 定义修饰键的常量,用于指定热键中包含的修饰键 public const uint MOD_ALT = 0x1; // Alt键 public const uint MOD_CONTROL = 0x2; // Ctrl键 public const uint MOD_SHIFT = 0x4; // Shift键 public const uint MOD_WIN = 0x8; // Windows键}步骤2:注册和注销热键接下来,我们需要在HotKeyManager类中添加方法来注册和注销热键。这些方法将调用我们之前定义的API函数。
public static void RegisterHotKey(Form f, int id, uint modKey, uint key){ if (!RegisterHotKey(f.Handle, id, modKey, key)) { throw new InvalidOperationException("Couldn't register the hot key."); }}public static void UnregisterHotKey(Form f, int id){ UnregisterHotKey(f.Handle, id);}步骤3:处理WM_HOTKEY消息为了响应热键事件,我们需要在我们的窗体类中处理WM_HOTKEY消息。这通常意味着重载WndProc方法。
protected override void WndProc(ref Message m){ base.WndProc(ref m); if (m.Msg == HotKeyManager.WM_HOTKEY) { MessageBox.Show("热键被按下!"); }}步骤4:注册和使用全局热键最后,我们需要在我们的窗体中注册我们想要的热键,并确保在窗体关闭时注销它们。
public partial MainForm : Form{ private int hotKeyId = 1; // 热键ID public MainForm() { InitializeComponent(); } protected override void OnLoad(EventArgs e) { base.OnLoad(e); HotKeyManager.RegisterHotKey(this, hotKeyId, HotKeyManager.MOD_CONTROL | HotKeyManager.MOD_SHIFT, (uint)Keys.A); } protected override void OnFormClosing(FormClosingEventArgs e) { base.OnFormClosing(e); HotKeyManager.UnregisterHotKey(this, hotKeyId); }}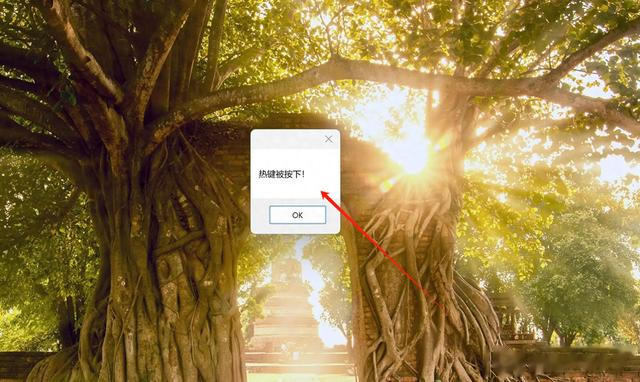
在这个示例中,我们注册了Ctrl + Shift + A作为全局热键。当然,你可以根据需要修改RegisterHotKey方法调用中的modKey和key参数来设置其他组合键。
总结通过上述步骤,你现在应该能够在自己的C#应用程序中实现全局热键功能了。这种技术可以在许多场景中提高用户体验,例如快速启动应用程序的特定功能或执行常用操作。只需确保选择不会与操作系统或其他应用程序冲突的热键组合。