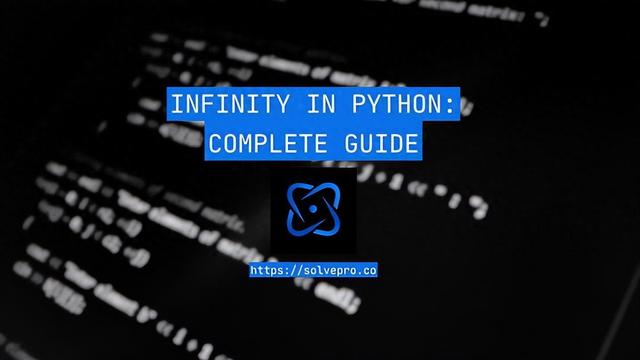
Python 中的 Infinity 不仅仅是一个理论概念,它还是一个帮助解决实际编程挑战的实用工具。让我们探索如何使用 Infinity 以及它在实际代码中的作用。
基本 Infinity作Python 提供了几种表示无穷大的方法:
# Using floatpositive_infinity = float('inf')negative_infinity = float('-inf')# Using math moduleimport mathmath_infinity = math.inf# Comparing with infinityprint(1e308 < float('inf')) # Output: Trueprint(-float('inf') < -1e308) # Output: True理解 Infinity 比较Infinity 有一些有趣的比较属性:
inf = float('inf')ninf = float('-inf')# Basic comparisonsprint(inf > 999999999999999) # Output: Trueprint(ninf < -999999999999999) # Output: True# Infinity with itselfprint(inf == inf) # Output: Trueprint(inf > inf) # Output: Falseprint(inf >= inf) # Output: True# Operations with infinityprint(inf + 1 == inf) # Output: Trueprint(inf - inf) # Output: nanprint(inf * 0) # Output: nan实际应用优先级队列from queue import PriorityQueueclass Task: def __init__(self, priority, description): self.priority = priority self.description = description def __lt__(self, other): # Lower number = higher priority return self.priority < other.prioritydef create_task_scheduler(): pq = PriorityQueue() # Add tasks with different priorities pq.put(Task(3, "Regular maintenance")) pq.put(Task(float('inf'), "Lowest priority task")) pq.put(Task(1, "Critical security update")) return pq# Usagescheduler = create_task_scheduler()while not scheduler.empty(): task = scheduler.get() print(f"Processing task: {task.description} (Priority: {task.priority})")最短路径算法def dijkstra(graph, start): distances = {node: float('inf') for node in graph} distances[start] = 0 unvisited = set(graph.keys()) while unvisited: # Find unvisited node with minimum distance current = min(unvisited, key=lambda node: distances[node]) if distances[current] == float('inf'): break unvisited.remove(current) for neighbor, weight in graph[current].items(): distance = distances[current] + weight if distance < distances[neighbor]: distances[neighbor] = distance return distances# Example usagegraph = { 'A': {'B': 4, 'C': 2}, 'B': {'A': 4, 'C': 1, 'D': 5}, 'C': {'A': 2, 'B': 1, 'D': 8}, 'D': {'B': 5, 'C': 8}}distances = dijkstra(graph, 'A')print(distances) # Shows shortest paths from A to all nodes使用窗口进行数据处理class SlidingWindowStats: def __init__(self, window_size=float('inf')): self.window_size = window_size self.values = [] self.sum = 0 def add_value(self, value): self.values.append(value) self.sum += value # Remove old values if window size exceeded if len(self.values) > self.window_size: self.sum -= self.values.pop(0) def get_average(self): if not self.values: return 0 return self.sum / len(self.values) def get_range(self): if not self.values: return 0 return max(self.values) - min(self.values)# Usagestats = SlidingWindowStats(window_size=3)for value in [1, 2, 3, 4, 5]: stats.add_value(value) print(f"Average: {stats.get_average():.2f}")使用无限 TTL 进行缓存from datetime import datetime, timedeltaclass Cache: def __init__(self, default_ttl=float('inf')): self.cache = {} self.default_ttl = default_ttl def set(self, key, value, ttl=None): expiry = datetime.now() + timedelta(seconds=ttl or self.default_ttl) self.cache[key] = { 'value': value, 'expires': expiry } def get(self, key): if key not in self.cache: return None item = self.cache[key] if item['expires'] != float('inf') and datetime.now() > item['expires']: del self.cache[key] return None return item['value']# Usagecache = Cache()cache.set('temp', 'temporary value', ttl=5) # Expires in 5 secondscache.set('permanent', 'never expires') # Never expires处理边缘情况使用 infinity 时,请小心某些作:
def safe_infinity_operations(a, b): """Handle common infinity operation edge cases.""" try: if math.isinf(a) and math.isinf(b): if a > 0 and b > 0: return float('inf') elif a < 0 and b < 0: return float('-inf') else: return float('nan') result = a + b if math.isnan(result): return "Operation results in NaN" return result except OverflowError: return "Operation causes overflow" except Exception as e: return f"Error: {str(e)}"# Test casesprint(safe_infinity_operations(float('inf'), float('inf')))print(safe_infinity_operations(float('inf'), float('-inf')))print(safe_infinity_operations(float('inf'), 100))性能注意事项def benchmark_infinity_comparisons(n): """Compare performance of infinity vs large numbers.""" import time inf = float('inf') large_num = 1e308 # Test infinity start = time.time() for _ in range(n): _ = 1 < inf inf_time = time.time() - start # Test large number start = time.time() for _ in range(n): _ = 1 < large_num large_num_time = time.time() - start return { 'infinity_time': inf_time, 'large_number_time': large_num_time }# Usageresults = benchmark_infinity_comparisons(1000000)print(f"Infinity comparisons: {results['infinity_time']:.6f} seconds")print(f"Large number comparisons: {results['large_number_time']:.6f} seconds")常见陷阱和解决方案def demonstrate_infinity_pitfalls(): inf = float('inf') # Division by infinity print(f"1/inf = {1/inf}") # Output: 0.0 # Infinity minus infinity try: result = inf - inf print(f"inf - inf = {result}") # Output: nan except Exception as e: print(f"Error: {e}") # Comparing NaN nan = float('nan') print(f"nan == nan: {nan == nan}") # Output: False print(f"nan is nan: {nan is nan}") # Output: False# Usagedemonstrate_infinity_pitfalls()了解如何在 Python 中使用 infinity 为许多实际编程挑战提供了解决方案。从实现算法到处理数据处理中的边缘情况,如果使用得当,Infinity 被证明是一个有用的工具。