哈喽,你好啊,我是雷工!
前面练习了按照组织类别查询某个组织的所有人员信息列表,接下来练习实现通过编号查询详细的人员信息;
以下为练习笔记。
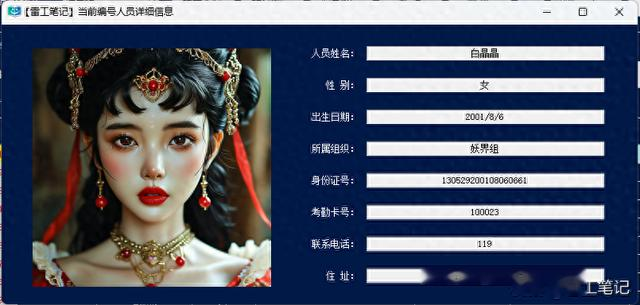
①当输入编号框为空时,点击【提交按钮】,提示:请输入要查询的人员编号!
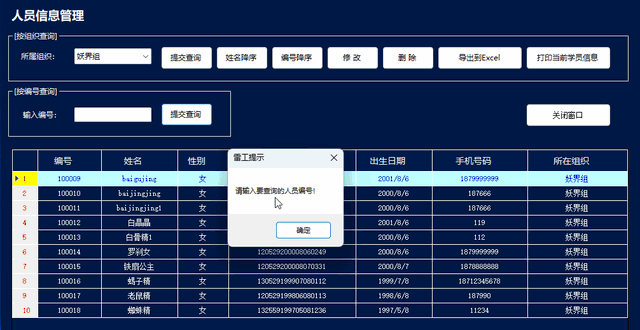
②当输入编号为非整数时,提示:输入编号必须是整数!
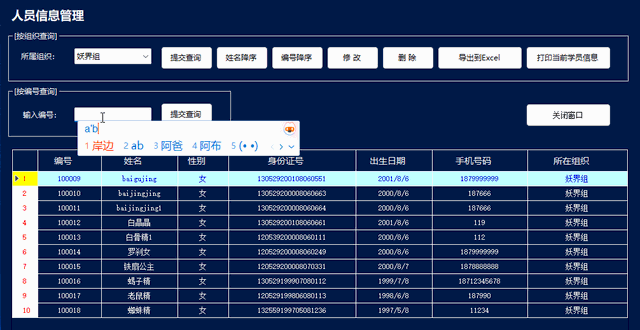
③当输入编号在系统中不存在时,提示:系统中不存在该编号信息!
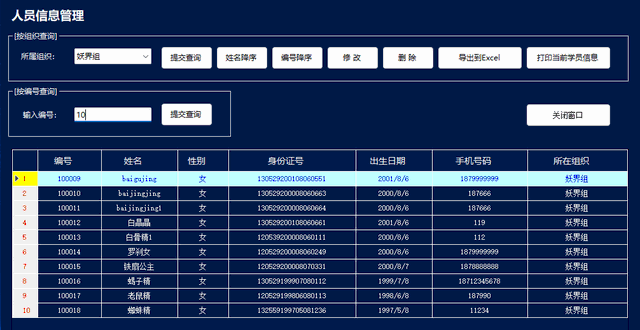
④当输入存在的正确编号时,在屏幕中间弹窗显示查询的人员信息。
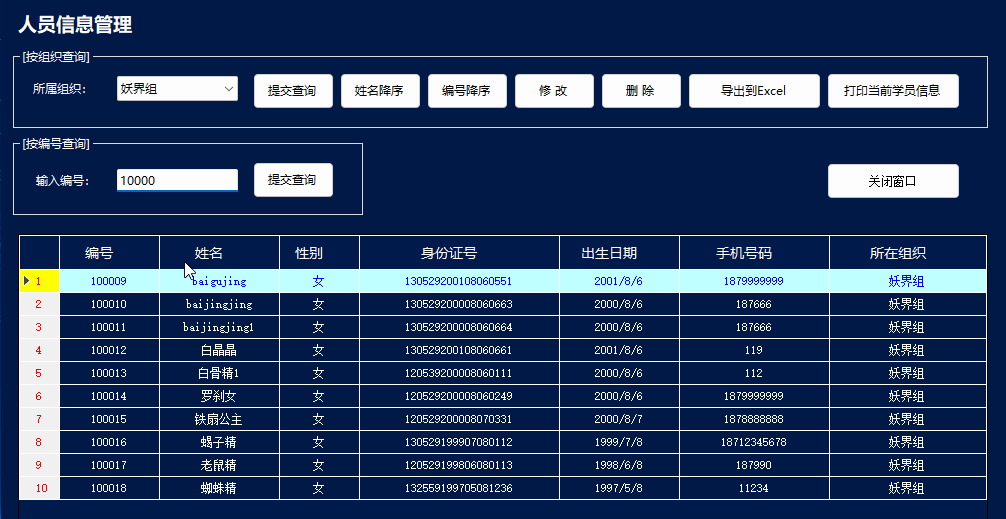
⑤当查询的人员信息在数据库中无图片时,显示根目录下的默认图
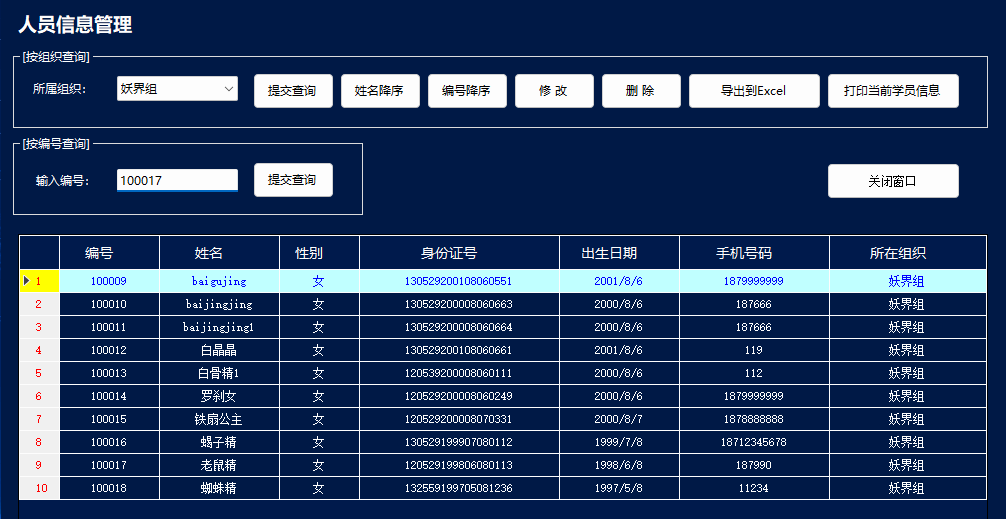
2.1、数据访问层
由于当前采用的是两层架构,所以首先从数据访问部分将查询需要的方法先写好;
在DAL中的人员信息访问类PeopleServer中添加根据编号查询人员信息时所需的方法:
代码如下:
/// <summary>/// 根据编号查询人员对象信息/// </summary>/// <param name="peopleId"></param>/// <returns></returns>public People GetPeopleById(string peopleId){ string sql = "select PeopleId,PeopleName,Gender,Birthday,PeopleIdNo,PhoneNumber,GroupName,PeopleAddress,CardNo,PeoImage from Peoples "; sql += "inner join Groups on Peoples.GroupId=Groups.GroupId "; sql += "where PeopleId={0}"; sql = string.Format(sql, peopleId); SqlDataReader objReader = SQLHelper.GetReader(sql); People objPeople = null; if(objReader.Read()) {objPeople = new People(){ PeopleId = Convert.ToInt32(objReader["PeopleId"]), PeopleName = objReader["PeopleName"].ToString(), Gender = objReader["Gender"].ToString(), PhoneNumber = objReader["PhoneNumber"].ToString(), Birthday = Convert.ToDateTime(objReader["Birthday"].ToString()), IdNumber = objReader["PeopleIdNo"].ToString(), GroupName = objReader["GroupName"].ToString(), CarNo = objReader["CardNo"].ToString(), Address = objReader["PeopleAddress"].ToString(), PeoImage = objReader["PeoImage"] == null ? "" : objReader["PeoImage"].ToString()}; } objReader.Close(); return objPeople;}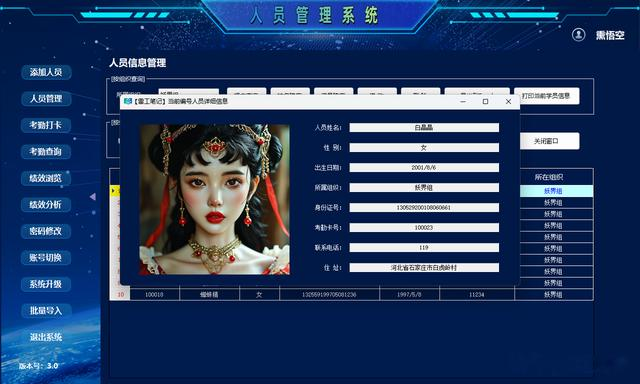
2.2、编号查询
根据编号查询时,首先在UI界面的对应按钮上双击,生成对应的按钮事件,然后在事件内编写详细的执行代码:
编写思路:
①首先验证编号输入框不为空;
②验证输入的编号为整数;
//根据人员编号查询private void btnQueryById_Click(object sender, EventArgs e){ //非空验证 if(this.txtPeopleId.Text.Trim().Length==0) { MessageBox.Show("请输入要查询的人员编号!", "雷工提示"); this.txtPeopleId.Focus(); return; } //整数验证 if(!Common.DataValidate.IsInteger(this.txtPeopleId.Text.Trim())) { MessageBox.Show("输入编号必须是整数!", "雷工提示"); this.txtPeopleId.SelectAll(); this.txtPeopleId.Focus(); return; } People objPeople = objPeoServer.GetPeopleById(this.txtPeopleId.Text.Trim()); if(objPeople==null) { MessageBox.Show("系统中不存在该编号信息!", "雷工提示"); } else { //显示查询编号对应人员的详细信息 FrmPeopleInfo objFrm = new FrmPeopleInfo(objPeople); objFrm.Show(); }}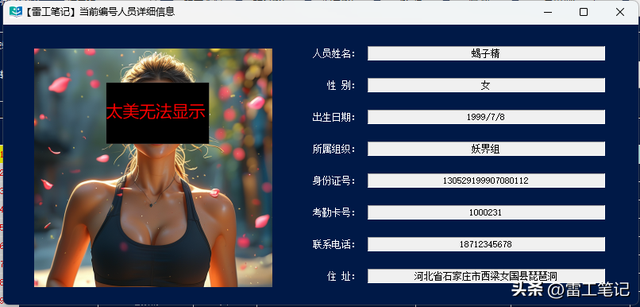
2.3、构造方法
当查询结果在另一个窗体展示时,可以通过构造方法将参数进行传递,此处人员信息较多,可传递人员对象;
①在FrmPeopleInfo窗体的代码中引入命名空间:
using Models;②添加带参数的构造方法
public FrmPeopleInfo(People objPeople):this(){ //显示人员详细信息 this.lblPeopleName.Text = objPeople.PeopleName; this.lblPeopleIdNo.Text = objPeople.IdNumber; this.lblPhoneNumber.Text = objPeople.PhoneNumber; this.lblBirthday.Text = objPeople.Birthday.ToShortDateString(); this.lblAddress.Text = objPeople.Address; this.lblGroup.Text = objPeople.GroupName; this.lblGender.Text = objPeople.Gender; this.lblCardNo.Text = objPeople.CarNo; //显示照片 this.pbPeo.Image = objPeople.PeoImage.Length != 0 ? (Image)new Common.SerializeObjectToString().DeserializeObject(objPeople.PeoImage) : Image.FromFile("default.png");}其中照片存储到数据库时将其序列化成二进制字符串,当从数据库读取显示时用到反序列化,将二进制字符串转换成图片
//将二进制序列字符串转换为Object类型对象public object DeserializeObject(string str){ IFormatter formatter = new BinaryFormatter(); byte[] byt = Convert.FromBase64String(str); object obj = null; using (Stream stream = new MemoryStream(byt, 0, byt.Length)) {obj = formatter.Deserialize(stream); } return obj;}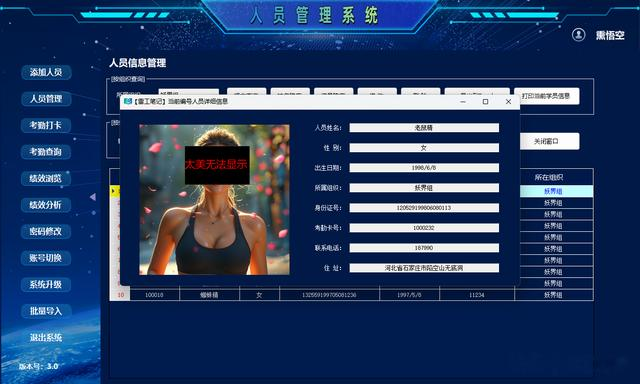
以上为按编号精准查询展示人员信息的实现,
你在实际项目中有用到过类似的功能么?
欢迎在留言区或交流群探讨。