1. 引言
多线程编程和并发技术是C语言中处理多任务和高性能计算的重要手段。它们允许程序同时执行多个任务,提高资源利用率和响应速度。本文将深入探讨C语言中多线程编程和并发的技术精髓,并通过实用的代码案例帮助读者深入理解。
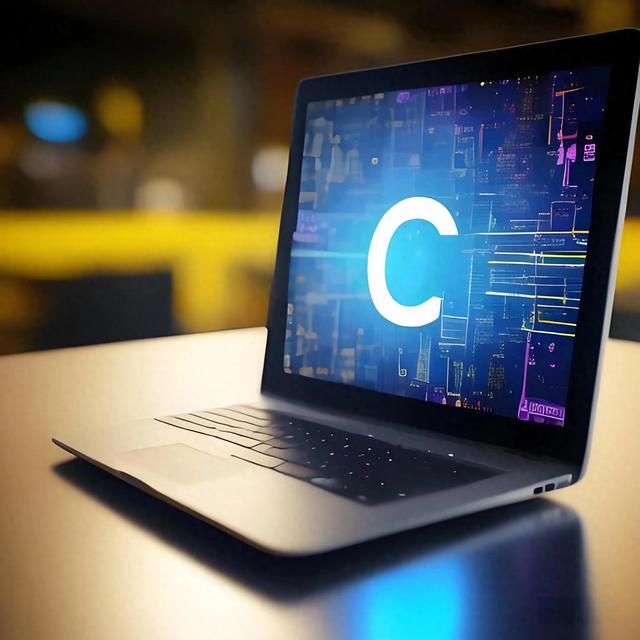
2. 线程创建与管理
2.1 技术精髓
C语言中的多线程编程通常依赖于POSIX线程(pthread)库,它提供了一组线程相关的API。通过这些API,我们可以创建、同步和管理线程。
2.2 代码案例
#include <stdio.h>#include <pthread.h>void* threadFunction(void* arg) { printf("Hello from the thread!\n"); return NULL;}int main() { pthread_t thread; if (pthread_create(&thread, NULL, threadFunction, NULL) != 0) { printf("Error creating thread.\n"); return 1; } pthread_join(thread, NULL); printf("Thread has finished.\n"); return 0;}这个例子中,我们使用pthread_create函数创建了一个新的线程,并指定了线程函数threadFunction。然后,我们使用pthread_join函数等待线程结束。这是多线程编程的基础,用于实现并行执行。
3. 线程同步
3.1 技术精髓
线程同步是并发编程中的关键问题,用于控制多个线程对共享资源的访问。互斥锁(mutex)、条件变量(condition variable)和读写锁(read-write lock)是常见的同步机制。
3.2 代码案例
#include <stdio.h>#include <pthread.h>pthread_mutex_t lock;int counter = 0;void* incrementCounter(void* arg) { for (int i = 0; i < 10000; i++) { pthread_mutex_lock(&lock); counter++; pthread_mutex_unlock(&lock); } return NULL;}int main() { pthread_t thread1, thread2; pthread_mutex_init(&lock, NULL); pthread_create(&thread1, NULL, incrementCounter, NULL); pthread_create(&thread2, NULL, incrementCounter, NULL); pthread_join(thread1, NULL); pthread_join(thread2, NULL); printf("Counter: %d\n", counter); pthread_mutex_destroy(&lock); return 0;}这个例子中,我们使用互斥锁pthread_mutex来保护共享资源counter。两个线程尝试同时增加counter的值,互斥锁确保了同时只有一个线程能够访问共享资源。这是线程同步的基础,用于避免竞态条件和数据不一致。
4. 线程通信
4.1 技术精髓
线程间通信是并发编程中另一个重要方面,它允许线程交换数据和状态信息。常见的通信机制包括共享内存、消息队列和信号量。
4.2 代码案例
#include <stdio.h>#include <pthread.h>pthread_mutex_t lock;pthread_cond_t condition;int dataReady = 0;void* producerThread(void* arg) { pthread_mutex_lock(&lock); dataReady = 1; pthread_cond_signal(&condition); pthread_mutex_unlock(&lock); return NULL;}void* consumerThread(void* arg) { pthread_mutex_lock(&lock); while (dataReady == 0) { pthread_cond_wait(&condition, &lock); } printf("Data is ready.\n"); pthread_mutex_unlock(&lock); return NULL;}int main() { pthread_t producer, consumer; pthread_mutex_init(&lock, NULL); pthread_cond_init(&condition, NULL); pthread_create(&producer, NULL, producerThread, NULL); pthread_create(&consumer, NULL, consumerThread, NULL); pthread_join(producer, NULL); pthread_join(consumer, NULL); pthread_mutex_destroy(&lock); pthread_cond_destroy(&condition); return 0;}这个例子中,我们使用条件变量pthread_cond来实现线程间的通信。生产者线程设置dataReady标志并通知消费者线程,而消费者线程等待dataReady标志变为1。这是线程通信的基础,用于协调线程间的操作和状态。
5. 总结
多线程编程和并发技术是C语言中处理多任务和高性能计算的重要手段。通过深入理解线程创建与管理、线程同步以及线程通信的技术精髓,我们可以更好地利用C语言实现高效的并发程序设计。掌握这些技术精髓不仅有助于提高编程技能,还能为学习更高级的并发编程模型和算法打下坚实的基础。