列表推导式提供了一种强大而优雅的方式来动态创建列表。
例:
squares = [x**2 for x in range(10)] # Squares of numbers 0-9print(squares) # Output: [0, 1, 4, 9, 16, 25, 36, 49, 64, 81]代码输出
[0, 1, 4, 9, 16, 25, 36, 49, 64, 81]列表推导式取代了手动循环和 append 调用的需要,使代码更简洁、更具表现力。
下面还有几个例子来说明列表推导的强大功能:
1.1 筛选列表
numbers = [1, 2, 3, 4, 5, 6]even_numbers = [num for num in numbers if num % 2 == 0]print(even_numbers) # Output: [2, 4, 6]代码输出
[2, 4, 6]1.2 嵌套列表推导
matrix = [[1, 2], [3, 4], [5, 6]]flattened_matrix = [num for row in matrix for num in row]print(flattened_matrix) # Output: [1, 2, 3, 4, 5, 6]代码输出
[1, 2, 3, 4, 5, 6]1.3 与函数结合
words = ["hello", "world", "Python"]uppercase_words = [word.upper() for word in words]print(uppercase_words) # Output: ['HELLO', 'WORLD', 'PYTHON']代码输出
['HELLO', 'WORLD', 'PYTHON']1.4 推导式中的条件逻辑
numbers = [-2, 5, 0, 1, 3]positives_and_zero = [num if num >= 0 else "negative" for num in numbers]print(positives_and_zero) # Output: ['negative', 5, 0, 1, 3]代码输出
['negative', 5, 0, 1, 3]2. 用于迭代多个列表的“zip”函数该 zip 函数将来自多个列表或可迭代对象的项目配对,从而可以轻松地同时遍历它们。
例:
names = ["Alice", "Bob", "Charlie"]ages = [25, 30, 28]for name, age in zip(names, ages): print(f"{name} is {age} years old")代码输出
Alice is 25 years oldBob is 30 years oldCharlie is 28 years oldzip 简化了涉及比较或组合多个列表中相应位置的元素的操作。
下面是另一个示例来说明该 zip 函数的多功能性:
2.1 创建词典
问题:有表示键和值的单独列表,并希望将它们合并到字典中。
法典:
keys = ["name", "age", "city"]values = ["Alice", 30, "New York"]person_dict = dict(zip(keys, values))print(person_dict) # Output: {'name': 'Alice', 'age': 30, 'city': 'New York'}代码输出
{'name': 'Alice', 'age': 30, 'city': 'New York'}3. 用于序列操作的切片Python 的切片语法提供了一种简洁的方法来提取列表、字符串或其他序列的子部分。
例:
numbers = [1, 2, 3, 4, 5]# Get the first three elementsfirst_three = numbers[:3] # Get the last two elementslast_two = numbers[-2:] # Reverse the listreversed_list = numbers[::-1]切片可以很容易地抓取序列的各个部分,反转它们,并以紧凑的方式执行其他操作。
以下是更多示例:
复制列表
问题:需要创建列表的副本,而不仅仅是对同一列表的引用。法典:
my_list = [1, 2, 3, 4]copy_of_list = my_list[:] # Slice from beginning to endcopy_of_list[0] = 10 print(my_list) # Output: [1, 2, 3, 4] print(copy_of_list) # Output: [10, 2, 3, 4]代码输出
[1, 2, 3, 4][10, 2, 3, 4]4. 使用索引进行循环的“枚举”函数该 enumerate 函数允许您在循环访问列表时访问元素的索引和值。
例:
colors = ["red", "green", "blue"]for index, color in enumerate(colors): print(f"{index}: {color}")代码输出
0: red1: green2: blue: enumerate 让您无需手动跟踪循环中的索引,从而使您的代码更简洁。
下面是利用该 enumerate 函数的更高级示例:
高级示例:在迭代时修改列表
问题:有时可能需要直接在循环中修改列表的元素。为此,如果修改更改了列表的长度或索引计算不正确,则使用传统的基于索引的循环可能会导致意外行为。法典:
words = ["hello", "good", "world", "nice"]for index, word in enumerate(words): if len(word) < 5: words[index] = word.upper() # Safely modify in-placeprint(words)代码输出
['hello', 'GOOD', 'world', 'NICE']5. 三元条件表达式三元表达式提供了一种编写简单条件赋值的紧凑方法。
例:
age = 25status = "Eligible" if age >= 18 else "Not eligible"print(status) # Output: Eligible代码输出
Eligible三元表达式可以使你的代码更简洁,特别是对于简单的 if-else 赋值。
下面是使用三元条件表达式的一种稍微高级的方法:
问题:您需要根据需要连续检查的多个条件做出决定。num = 25result = "Greater than 30" if num > 30 else "Greater than 10" if num > 10 else "Less than or equal to 10"print(result) # Output: Greater than 10代码输出
Greater than 10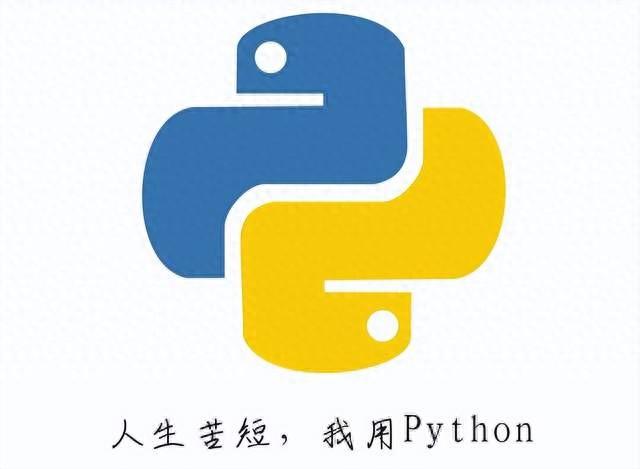