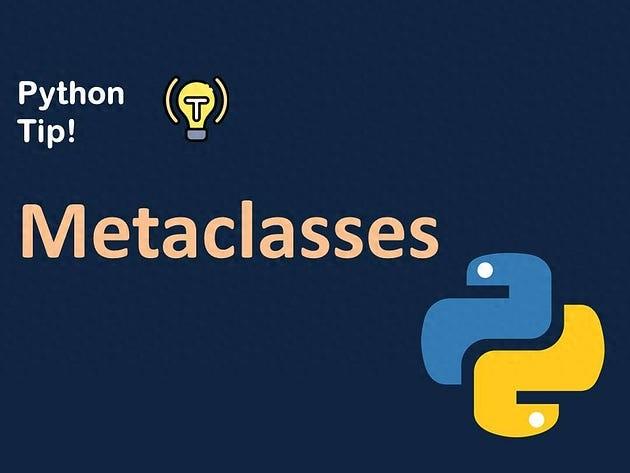
Python 是一种用途广泛且功能强大的编程语言,以其简单性和可读性而闻名。但是,它的一些高级功能(如元类)乍一看似乎令人生畏。在这篇文章中,我们将揭开元类的神秘面纱,探索它们的用途,并通过实际示例来帮助你了解它们是如何工作的。
什么是元类?在 Python 中,一切都是一个对象,包括类。类的类型称为元类。默认情况下,大多数类的元类是 type。元类允许您控制类的创建和行为,使其成为高级 Python 编程的强大工具。将元类视为 “创建类的蓝图”。正如类定义其实例的行为一样,元类定义类的行为。
type()函数type() 函数是元类的核心。它有两个用途:
检查对象的类型:当与一个参数一起使用时,它将返回对象的类型。动态创建类:当与三个参数一起使用时,它会创建一个新类。让我们先来探索一下 type() 是如何工作的。
1. 检查对象的类型print(type(1)) # <class 'int'> - Type of integerprint(type(1.0)) # <class 'float'> - Type of floatdef func(): passprint(type(func)) # <class 'function'> - Type of functionclass MyClass: passprint(type(MyClass)) # <class 'type'> - Type of (metaclass is `type`)在这里,我们看到类的类型是 type,这是默认的元类。
2. 动态创建类你可以使用 type() 动态创建类。
type(class_name, base_classes, attributes)class_name:类的名称(字符串)。base_classes:基类的元组(用于继承)。attributes:属性(方法和变量)的字典。示例 1:创建基本类TestCls = type('TestCls', (), {}) # No basees or attributesprint(type(TestCls)) # <class 'type'> - Type of dynamically created示例 2:添加属性TestCls = type('TestCls', (), {'x': 1, 'y': 2})print(f'x = {TestCls.x}') # Accessing attribute xprint(f'y = {TestCls.y}') # Accessing attribute y3. 使用动态创建的类进行继承您还可以创建动态继承自另一个类的类。
# Baseclass Player: def show(self): print('This is Player')# Creating a dynamically with a base and attributesTestCls = type('TestCls', (Player,), {'x': 1, 'y': 2})obj = TestCls()obj.show() # Calling inherited method from Player4. 向动态创建的类添加方法您可以通过将方法包含在 attributes 字典中,将方法添加到动态创建的类中。
# Method to be added to thedef show(self): print('This is show function')# Creating a dynamically with a methodTestCls = type('TestCls', (), {'show': show})obj = TestCls()obj.show() # Calling the dynamically added method5. 在类外部添加属性您可以在创建对象后向对象添加属性。
# Method to be added to thedef show(self): print('This is show function')# Creating a dynamicallyTestCls = type('TestCls', (), {'show': show})obj = TestCls()# Adding attributes to the objectobj.x = 1 # Adding attribute x to objectobj.name = "Test Class" # Adding attribute name to objectprint(f'x = {obj.x}') # Accessing attribute xprint(f'name = {obj.name}') # Accessing attribute name自定义元类您可以通过子类化 type 创建自定义元类。这允许您自定义类的创建和行为。
# Custom metaclassclass Meta(type): def __new__(self, name, bases, attrs): # Modify the creation process print(f'Creating {name} with metaclass {self}') return type(name, bases,attrs)# Using the custom metaclassclass MyClass(metaclass=Meta): pass示例:使用 MetaClass 的单例模式# singleton metaclassclass SingletonMeta(type): __instances = {} def __call__(cls,*args, **kwargs): if cls not in cls.__instances: cls.__instances[cls] = super(SingletonMeta, cls).__call__(*args, **kwargs) return cls.__instances[cls] # Using the singleton metaclassclass SingletonClass(metaclass=SingletonMeta): def __init__(self, value): self.value = value # Testing the singletonobj1 = SingletonClass(10) # This will create a new object of SingletonClassobj2 = SingletonClass(20) # This will not create a new object but return the existing oneprint(obj1.value) # Output: 10print(obj2.value) # Output: 10 - Same value as obj1print(obj1 == obj2) # Output: True什么时候应该使用元类?元类功能强大,但它们会使您的代码更加复杂和难以理解。仅在绝对必要时使用它们,例如在构建框架或库时。对于大多数日常编程,常规类和继承就足够了。
Youtube 视频
结论元类是 Python 的一个引人入胜的高级功能,它允许您控制类的创建和行为。虽然它们乍一看可能看起来很复杂,但理解它们可以让你更深入地了解 Python 的工作原理。
在这篇文章中,探讨了:
元类和 type() 函数的基础知识。如何动态创建类。向动态创建的类添加属性和方法。创建自定义元类及其实际用例。