在 C 语言中,errno 是一个全局变量,用于存储最近发生的系统调用或库函数调用的错误代码。正确使用 errno 可以帮助开发者诊断程序中的错误并采取适当的措施。下面是如何正确使用 errno 的指南:
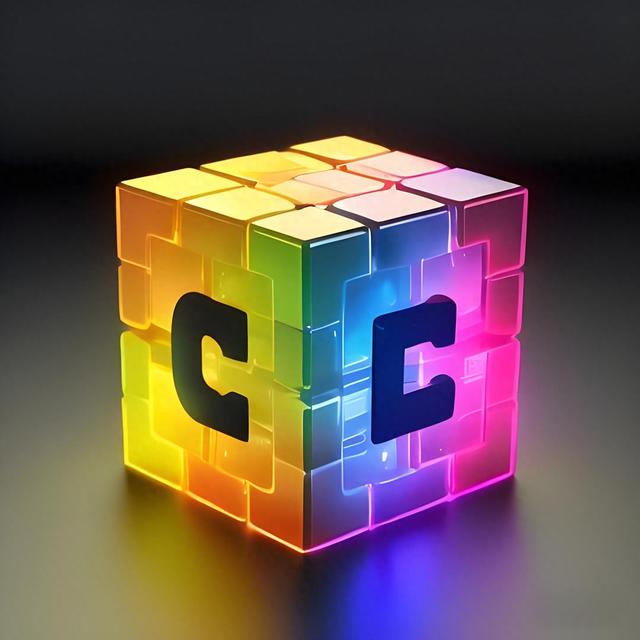
首先,需要包含 <errno.h> 头文件,它定义了 errno 变量以及其他与错误相关的宏和常量。
1#include <errno.h>2. 检查函数调用的结果在调用可能导致错误的函数之后,应当检查函数的返回值,判断是否出现了错误。例如,打开文件的 open() 函数,如果返回 -1 表示发生错误。
1int fd = open("example.txt", O_RDONLY);2if (fd == -1) {3 // 出现错误4}3. 保存errno在检查到错误之后,应当立即读取 errno 的值。这是因为 errno 是一个全局变量,可能会被随后的系统调用覆盖。
1if (fd == -1) {2 int saved_errno = errno;3 // 处理错误4}4. 使用perror打印错误信息perror 函数可以用来打印一个描述错误的字符串,通常用于调试目的。
1if (fd == -1) {2 perror("Failed to open file");3}5. 使用strerror获取错误描述strerror 函数可以将 errno 的值转换为人类可读的错误描述字符串。
1if (fd == -1) {2 const char *error_str = strerror(errno);3 printf("Failed to open file: %s\n", error_str);4}6. 使用errno宏errno 宏可以帮助你确定具体的错误原因。例如,EACCES 表示权限错误,ENOENT 表示找不到文件等。
1if (fd == -1) {2 if (errno == EACCES) {3 printf("Permission denied\n");4 } else if (errno == ENOENT) {5 printf("File not found\n");6 } else {7 printf("Other error: %s\n", strerror(errno));8 }9}7. 清理errno在处理完错误后,可以显式地将 errno 设置为 0,以清除之前的错误状态。
1if (fd == -1) {2 // 处理错误3 errno = 0; // 清除错误状态4}示例代码下面是一个完整的示例,展示了如何使用 errno 来处理文件打开错误:
1#include <stdio.h>2#include <errno.h>3#include <string.h>4#include <fcntl.h>56int main() {7 int fd = open("example.txt", O_RDONLY);8 if (fd == -1) {9 int saved_errno = errno;10 const char *error_str = strerror(saved_errno);11 printf("Failed to open file: %s\n", error_str);1213 if (saved_errno == EACCES) {14 printf("Permission denied\n");15 } else if (saved_errno == ENOENT) {16 printf("File not found\n");17 } else {18 printf("Other error: %s\n", error_str);19 }20 } else {21 // 文件成功打开,可以进行其他操作22 close(fd);23 }2425 return 0;26}注意事项errno 的值可能会被随后的系统调用覆盖,所以在检查错误后尽快读取 errno 的值。不要依赖 errno 的值来决定程序的流程控制,而应该将其用于诊断和记录错误。在多线程程序中,errno 的值可能会被其他线程更改,因此在多线程环境下使用 errno 时要特别小心。通过正确使用 errno,你可以更好地理解和处理 C 语言程序中的错误情况。