引言
C语言作为一门经典的编程语言,自1972年由Dennis Ritchie在AT&T贝尔实验室设计以来,凭借其简洁、高效、灵活的特点,在操作系统、嵌入式系统、系统软件等领域得到了广泛的应用。本文将探讨C语言的一些高级技巧及其在实际编程中的应用,帮助读者更深入地理解和掌握这门语言。
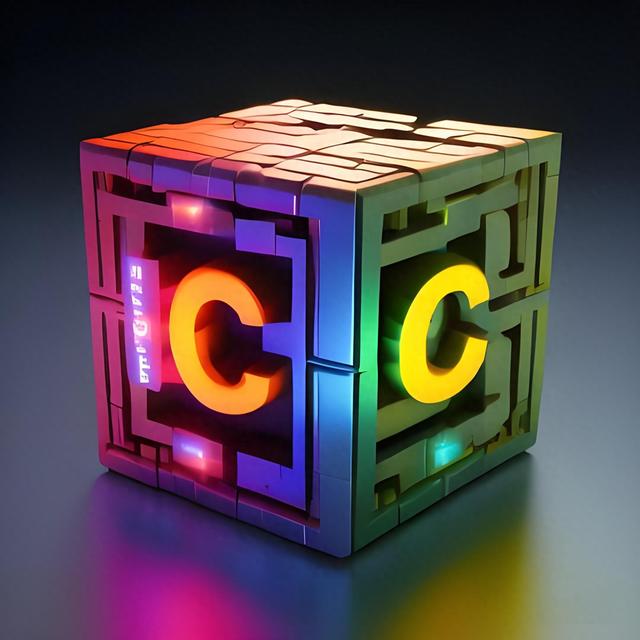
1. 指针与内存管理
C语言的核心特性之一是对指针的直接操作。指针允许程序员直接访问和操作内存,极大地提高了程序的灵活性和效率。然而,这也带来了一定的风险,如内存泄漏、野指针等问题。
1.1 动态内存分配
C语言中,动态内存分配主要通过malloc、calloc、realloc和free四个函数来实现。使用这些函数时,需要注意内存分配成功与否的检查、内存释放以及避免内存泄漏等问题。
#include <stdio.h>#include <stdlib.h>int main() { int *p = (int *)malloc(10 * sizeof(int)); if (p == NULL) { fprintf(stderr, "Memory allocation failed\n"); exit(EXIT_FAILURE); } // 使用分配的内存 for (int i = 0; i < 10; ++i) { p[i] = i; } // 释放内存 free(p); p = NULL; return 0;}1.2 指针运算与数组
C语言中,指针和数组紧密相关。指针可以用于访问数组元素,也可以进行指针算术运算。
#include <stdio.h>int main() { int arr[10] = {0, 1, 2, 3, 4, 5, 6, 7, 8, 9}; int *p = arr; for (int i = 0; i < 10; ++i) { printf("%d ", *(p + i)); // 等同于 printf("%d ", arr[i]); } printf("\n"); return 0;}2. 函数指针与回调函数
函数指针是C语言中的一种高级特性,它允许将函数作为参数传递给其他函数,或者将函数作为返回值。这一特性在实现回调函数、事件驱动编程等方面非常有用。
#include <stdio.h>// 函数声明void print(int);int main() { // 函数指针 void (*func_ptr)(int) = print; // 使用函数指针调用函数 func_ptr(42); return 0;}// 函数定义void print(int num) { printf("Number: %d\n", num);}3. 结构体与联合体
C语言中的结构体(struct)和联合体(union)允许程序员定义复合数据类型,用于表示更复杂的数据结构。
3.1 结构体
结构体用于表示一组相关数据的集合,如表示一个学生的信息。
#include <stdio.h>// 定义结构体typedef struct { char name[50]; int age; float score;} Student;int main() { // 创建结构体变量 Student stu = {"Alice", 20, 90.5}; // 访问结构体成员 printf("Name: %s\nAge: %d\nScore: %.1f\n", stu.name, stu.age, stu.score); return 0;}3.2 联合体
联合体用于表示一组不同类型的数据,但只能同时存储其中一个成员的数据。
#include <stdio.h>// 定义联合体typedef union { int i; float f; char str[20];} Data;int main() { Data data; // 存储整型数据 data.i = 42; printf("Integer: %d\n", data.i); // 存储浮点型数据 data.f = 3.14; printf("Float: %.2f\n", data.f); // 存储字符串数据 strcpy(data.str, "Hello"); printf("String: %s\n", data.str); return 0;}4. 链表
链表是一种常见的数据结构,它通过指针将一系列数据节点连接起来。C语言中的链表通常使用动态内存分配来实现。
#include <stdio.h>#include <stdlib.h>// 定义链表节点结构体typedef struct Node { int data; struct Node *next;} Node;int main() { // 创建链表节点 Node *head = (Node *)malloc(sizeof(Node)); if (head == NULL) { fprintf(stderr, "Memory allocation failed\n"); exit(EXIT_FAILURE); } head->data = 1; head->next = NULL; // 添加新节点 Node *current = head; for (int i = 2; i <= 5; ++i) { Node *new_node = (Node *)malloc(sizeof(Node)); if (new_node == NULL) { fprintf(stderr, "Memory allocation failed\n"); exit(EXIT_FAILURE); } new_node->data = i; new_node->next = NULL; current->next = new_node; current = new_node; } // 遍历链表并打印数据 current = head; while (current != NULL) { printf("%d ", current->data); current = current->next; } printf("\n"); // 释放链表内存 current = head; while (current != NULL) { Node *temp = current; current = current->next; free(temp); } return 0;}5. 文件操作
C语言提供了丰富的文件操作函数,如fopen、fclose、fprintf、fscanf等,用于对文件进行读写操作。
#include <stdio.h>int main() { // 打开文件 FILE *fp = fopen("example.txt", "w+"); if (fp == NULL) { perror("Error opening file"); return -1; } // 写入数据 fprintf(fp, "Hello, World!\n"); // 重置文件指针 rewind(fp); // 读取数据 char buffer[100]; fgets(buffer, 100, fp); printf("%s", buffer); // 关闭文件 fclose(fp); return 0;}6. 系统调用与进程控制
C语言提供了与操作系统交互的能力,包括系统调用和进程控制。这些功能使得C语言成为开发操作系统、系统软件和嵌入式系统的理想选择。
#include <stdio.h>#include <stdlib.h>#include <unistd.h>#include <sys/types.h>#include <sys/wait.h>int main() { // 创建子进程 pid_t pid = fork(); if (pid < 0) { perror("Fork failed"); exit(EXIT_FAILURE); } else if (pid == 0) { // 子进程执行 printf("Child process\n"); exit(EXIT_SUCCESS); } else { // 父进程执行 int status; waitpid(pid, &status, 0); printf("Parent process\n"); } return 0;}总结
本文介绍了C语言的一些高级技巧和应用,包括指针与内存管理、函数指针与回调函数、结构体与联合体、链表、文件操作以及系统调用与进程控制。这些知识点都是C语言编程中不可或缺的部分,掌握它们对于深入理解和运用C语言至关重要。希望读者能够通过本文的学习,对C语言有更深入的了解,并在实际编程中能够灵活运用这些技巧。
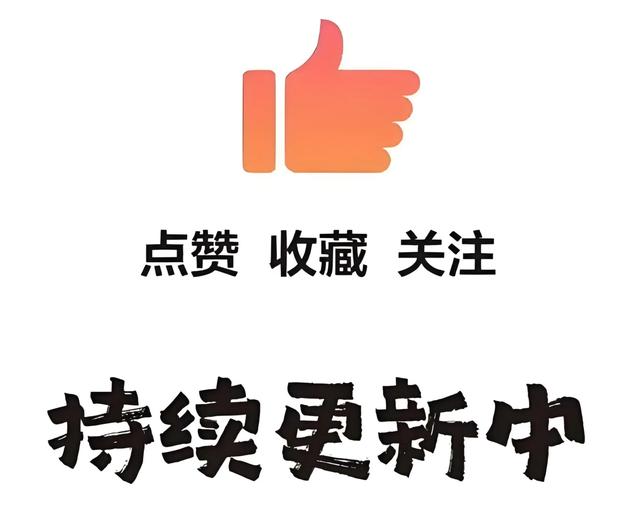