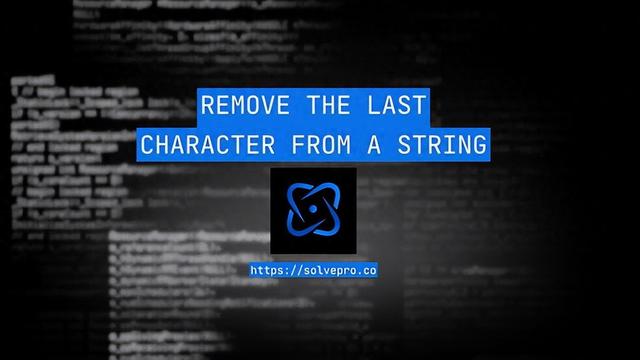
使用字符串是 Python 编程的基本部分,一个常见任务是从字符串中删除最后一个字符。无论您是清理用户输入、处理文本文件还是处理数据,了解此任务的不同方法都可以使您的代码更加高效和可读。
快速解决方案:字符串切片从字符串中删除最后一个字符的最快、最易读的方法是使用 Python 的切片表示法:
text = "Hello World!"result = text[:-1] # Returns "Hello World"这种方法干净高效 — 它会创建一个新字符串,其中包含除最后一个字符之外的所有内容。'-1' 索引告诉 Python 在结束之前停止一个字符。
五种不同的方法(以及何时使用每种方法)1. 字符串切片 — 通用解决方案字符串切片在所有场景中都能可靠地工作:
# Basic slicingword = "Python!"without_last = word[:-1] # Returns "Python"# Works with empty stringsempty = ""result = empty[:-1] # Returns "" (empty string)# Works with single characterssingle = "A"result = single[:-1] # Returns "" (empty string)字符串切片是内存高效的,因为 Python 在后台优化了字符串切片。它也非常可读 — 即使是新的 Python 开发人员也可以快速理解 '[:-1]' 的作用。
2. 使用 slice() — 当您需要更多控制时当你需要存储或重用切片模式时,'slice()' 函数提供了更大的灵活性:
# Creating a reusable sliceremove_last = slice(None, -1)text = "Hello World!"result = text[remove_last] # Returns "Hello World"# Reuse the same slice on different stringsnames = ["John!", "Mary!", "Steve!"]clean_names = [name[remove_last] for name in names]# Returns ['John', 'Mary', 'Steve']当你在不同的琴弦上重复应用相同的切片模式,或者当你需要动态修改切片参数时,这种方法会很出色。
3. 使用 rsplit() 进行字符串作 — 用于基于模式的删除有时,仅当最后一个字符与特定模式匹配时,才需要删除最后一个字符:
# Remove last character if it's a specific punctuation markdef remove_last_if_punctuation(text, punct='.!?'): if text and text[-1] in punct: return text[:-1] return text# Examplesprint(remove_last_if_punctuation("Hello!")) # Returns "Hello"print(remove_last_if_punctuation("Hello")) # Returns "Hello" (unchanged)print(remove_last_if_punctuation("Hi...")) # Returns "Hi.."当您清理文本数据并且需要选择性地选择删除的内容时,此方法非常完美。
4. 使用 removesuffix() (Python 3.9+) — 用于特定结尾如果你使用的是 Python 3.9 或更高版本,'removesuffix()' 非常适合删除特定的结尾:
# Remove specific endingstext = "filename.txt"result = text.removesuffix('.txt') # Returns "filename"# Only removes if it exactly matchesemail = "user.name@email.com"result = email.removesuffix('com') # Returns "user.name@email."result = email.removesuffix('.com') # Returns "user.name@email"# Doesn't modify if suffix doesn't matchtext = "Hello World!"result = text.removesuffix('?') # Returns "Hello World!" (unchanged)在处理文件名、URL 或任何具有标准化结尾的文本时,此方法特别有用。
5. 正则表达式 — 用于复杂模式匹配当您需要根据模式删除更复杂的最后一个字符时:
import redef remove_last_matching(text, pattern=r'[^a-zA-Z]'): """Remove last character if it matches the given pattern.""" if text and re.match(pattern, text[-1]): return text[:-1] return text# Examplesprint(remove_last_matching("Hello123", r'\d')) # Returns "Hello12"print(remove_last_matching("Hello!!!", r'[!]')) # Returns "Hello!!"print(remove_last_matching("Hello", r'\d')) # Returns "Hello" (unchanged)当您需要根据复杂模式或多个条件删除最后一个字符时,正则表达式是理想的选择。
您真正想阅读的作者的笔记嘿,我是 Ryan 。我希望您发现这篇文章有用!
我只是想告诉你我在经历了太多次深夜调试会议后构建的东西。
事实是这样的:我厌倦了花费数小时寻找错误,滚动浏览无休止的 Stack Overflow 线程,并获得实际上并不能解决我问题的通用 AI 响应。
所以我构建了 SolvePro (https://solvepro.co/ai/),结果证明它是我希望几年前就拥有的工具。
认识 SolvePro:您的 Programming AI 合作伙伴还记得当你终于理解了一个概念,一切都只是点击时的那种感觉吗?
这就是我想创造的 — 不仅仅是另一个 AI 工具,而是一个真正的学习伴侣,可以帮助那些 “啊哈 ”的时刻更频繁地发生。
SolvePro 与其他 AI 的不同之处在于它如何指导您的学习之旅。根据您的编码问题和风格,它会推荐符合您需求的测验和真实项目。
实际应用清理 CSV 数据使用 CSV 文件时,您可能需要删除尾随分隔符:
def clean_csv_line(line): # Remove trailing comma if present return line[:-1] if line.endswith(',') else line# Example usageraw_data = ["John,Doe,Engineer,", "Jane,Smith,Designer,"]cleaned_data = [clean_csv_line(line) for line in raw_data]# Returns ['John,Doe,Engineer', 'Jane,Smith,Designer']处理日志文件解析日志文件时,您可能需要删除尾随的换行符或时间戳:
def clean_log_line(line): # Remove trailing newline and timestamp line = line.rstrip('\n') # Remove newline if line.endswith(']'): # Remove timestamp if present return line[:-21] # Standard timestamp format is 20 chars return line# Example usagelog_line = "User login successful [2024-03-21 15:30:45]"cleaned = clean_log_line(log_line) # Returns "User login successful"URL 清理使用 URL 时,您可能需要删除尾部斜杠:
def normalize_url(url): # Remove trailing slash if present return url[:-1] if url.endswith('/') else url# Example usageurls = [ "https://example.com/", "https://example.com/path/", "https://example.com/path"]normalized = [normalize_url(url) for url in urls]# Returns ['https://example.com', 'https://example.com/path', 'https://example.com/path']常见的陷阱以及如何避免它们空字符串处理# Wrong way - will raise IndexErrordef wrong_remove_last(text): return text[-2] # Crashes on empty strings or single characters# Right way - handles all casesdef safe_remove_last(text): return text[:-1] if text else ""Unicode 字符处理# Some unicode characters are multiple bytestext = "Hello" # World emojiprint(len(text)) # Returns 6, not 5!# Use string slicing - it handles unicode correctlyresult = text[:-1] # Returns "Hello"就地修改字符串# Wrong way - strings are immutabletext = "Hello!"text[-1] = "" # Raises TypeError# Right way - create new stringtext = "Hello!"text = text[:-1] # Creates new string "Hello"性能提示在循环中使用大型字符串或处理多个字符串时,请考虑以下性能优化:
# For multiple operations, join is more efficient than repeated concatenationsuffixes = ['!', '?', '.']text = "Hello!"# Less efficientfor suffix in suffixes: if text.endswith(suffix): text = text[:-1]# More efficientif text[-1] in suffixes: text = text[:-1]请记住,Python 字符串是不可变的,因此任何修改都会创建一个新字符串。在逐行处理大文件时,请考虑使用生成器来有效地管理内存:
def process_large_file(filename): with open(filename, 'r') as file: for line in file: yield line[:-1] # Remove last character from each line# Memory-efficient processingfor processed_line in process_large_file('large_file.txt'): # Process each line without loading entire file into memory pass通过了解这些不同的方法及其适当的使用案例,您可以选择最适合您特定需求的方法,同时保持代码干净、高效和可读。