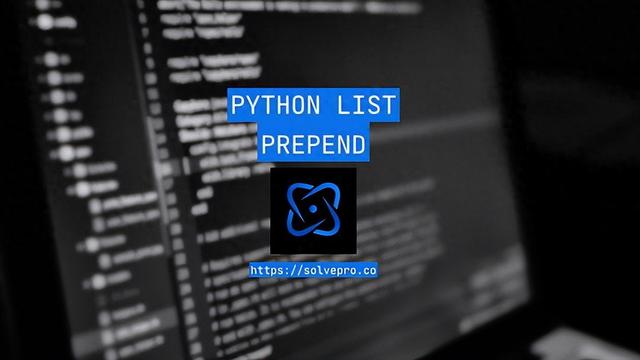
在 Python 中将元素添加到列表的开头似乎是一项基本操作,但它可能会对代码的性能和可读性产生重大影响。让我们深入研究将元素添加到 Python 列表的各种方法,并完成实际示例和性能注意事项。
prepend 在 Python 中是什么意思?Prepending 表示将一个或多个元素添加到列表的开头。虽然 Python 没有专用的 'prepend()' 方法,但它提供了几种方法来实现这一点:
numbers = [2, 3, 4]# Using insert(0, element)numbers.insert(0, 1) # [1, 2, 3, 4]# Using list concatenationnumbers = [0] + numbers # [0, 1, 2, 3, 4]让我们详细探讨每种方法,并了解何时使用哪种方法。
方法 1:使用 insert() — 直接方法'insert()' 方法是 Python 的内置方法,可以在列表中的任意位置添加元素,包括开头:
fruits = ['orange', 'banana', 'mango']fruits.insert(0, 'apple')print(fruits) # ['apple', 'orange', 'banana', 'mango']# You can also insert multiple timesfruits.insert(0, 'grape')print(fruits) # ['grape', 'apple', 'orange', 'banana', 'mango']何时使用 insert()- 当您需要添加单个元素时- 当代码可读性比性能更重要时- 当您使用小型列表时
重要的 insert() 详细信息# Insert works with any data typenumbers = [2, 3, 4]numbers.insert(0, 1.5) # [1.5, 2, 3, 4]numbers.insert(0, "one") # ["one", 1.5, 2, 3, 4]numbers.insert(0, [0, 0.5]) # [[0, 0.5], "one", 1.5, 2, 3, 4]# Insert returns None - common beginner mistakenumbers = numbers.insert(0, 1) # Wrong! numbers will be None方法 2:列表连接 — 简洁的方法使用 '+' 运算符连接列表是另一种常见的元素预置方法:
colors = ['blue', 'green']# Single element prependcolors = ['red'] + colorsprint(colors) # ['red', 'blue', 'green']# Multiple element prependcolors = ['purple', 'pink'] + colorsprint(colors) # ['purple', 'pink', 'red', 'blue', 'green']真实示例:构建事务历史记录这是一个实际示例,其中 prepending 很有用 — 维护事务历史记录:
class TransactionHistory: def __init__(self): self.transactions = [] def add_transaction(self, transaction): # New transactions go at the start - most recent first self.transactions.insert(0, { 'timestamp': transaction['time'], 'amount': transaction['amount'], 'type': transaction['type'] }) def get_recent_transactions(self, limit=5): return self.transactions[:limit]# Usage examplehistory = TransactionHistory()history.add_transaction({ 'time': '2024-03-15 10:30', 'amount': 50.00, 'type': 'deposit'})history.add_transaction({ 'time': '2024-03-15 14:20', 'amount': 25.00, 'type': 'withdrawal'})recent = history.get_recent_transactions()# Most recent transaction appears first方法 3:使用 deque 进行高效前置对于需要频繁添加到大型列表的情况下,'collections.deque' 是最好的选择:
from collections import deque# Create a deque from a listnumbers = deque([3, 4, 5])# Prepend single elementsnumbers.appendleft(2)numbers.appendleft(1)# Convert back to list if needednumbers_list = list(numbers)print(numbers_list) # [1, 2, 3, 4, 5]性能比较示例下面是一个比较不同 prepend 方法性能的实际示例:
import timefrom collections import dequedef measure_prepend_performance(size): # Regular list with insert start = time.time() list_insert = [] for i in range(size): list_insert.insert(0, i) list_time = time.time() - start # List concatenation start = time.time() list_concat = [] for i in range(size): list_concat = [i] + list_concat concat_time = time.time() - start # Deque start = time.time() d = deque() for i in range(size): d.appendleft(i) deque_time = time.time() - start return { 'insert': list_time, 'concat': concat_time, 'deque': deque_time }# Test with 10,000 elementsresults = measure_prepend_performance(10000)for method, time_taken in results.items(): print(f"{method}: {time_taken:.4f} seconds")处理边缘情况和常见错误让我们看看一些可能会让您绊倒的情况以及如何处理它们:
# 1. Prepending None or empty listsnumbers = [1, 2, 3]numbers.insert(0, None) # [None, 1, 2, 3] - Validnumbers = [] + numbers # [1, 2, 3] - Empty list has no effect# 2. Prepending to an empty listempty_list = []empty_list.insert(0, 'first') # Works fine: ['first']# 3. Type mixing - be careful!numbers = [1, 2, 3]numbers = ['1'] + numbers # ['1', 1, 2, 3] - Mixed types possible but risky# 4. Modifying list while iteratingnumbers = [1, 2, 3]for num in numbers: numbers.insert(0, num * 2) # Don't do this! Use a new list instead使用 List Prepend 的实用技巧以下是一些基于实际使用情况的具体提示:
# 1. Bulk prepending - more efficient than one at a timeold_items = [4, 5, 6]new_items = [1, 2, 3]combined = new_items + old_items # Better than multiple insert() calls# 2. Converting types safelystring_nums = ['1', '2', '3']numbers = []for num in string_nums: try: numbers.insert(0, int(num)) except ValueError: print(f"Couldn't convert {num} to integer")# 3. Maintaining a fixed-size listmax_size = 5recent_items = [3, 4, 5]recent_items.insert(0, 2)if len(recent_items) > max_size: recent_items.pop() # Remove last item if list too long何时选择每种方法下面是一个快速决策指南:
1. 在以下情况下使用 'insert(0, element)':— 您正在使用小型列表— 代码清晰是您的首要任务— 您只需偶尔预置
2. 在以下情况下使用列表连接 ('[element] + list'):— 您需要最清晰的语法— 您一次预置多个元素— 您需要链接操作
3. 在以下情况下使用 'deque':— 您正在处理大型列表— 性能至关重要— 您需要经常预置和预置
请记住,过早的优化是万恶之源 — 从最易读的解决方案 ('insert()') 开始,只有在性能成为真正问题时才切换到 'deque'。
通过掌握这些在 Python 列表中预置元素的不同方法,您将能够为每种特定情况选择合适的工具。关键是了解特定用例中可读性、性能和功能之间的权衡。