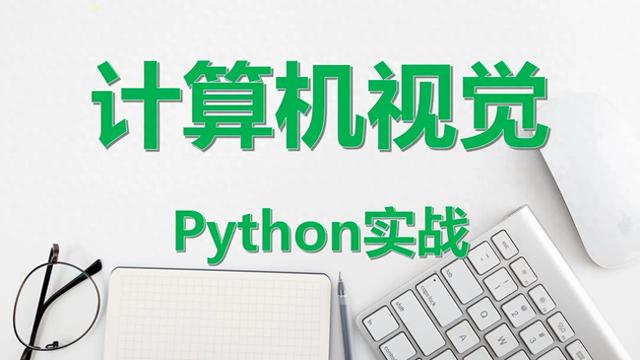
本文将介绍如何使用Python实现一个基本的人脸识别系统。我们将采用OpenCV库来处理图像和视频流,并利用dlib库中的预训练模型来进行人脸检测与特征点定位。此外,我们还会使用face_recognition库简化部分过程,以达到快速开发的目的。
环境配置安装必要的库
首先需要安装以下Python库:
pip install opencv-python-headless
pip install dlib
pip install face_recognition
确认依赖项
确认安装了正确的版本,并确保所有依赖项都已正确安装。
编程步骤
步骤 1: 导入必要的库
import cv2
import face_recognition
步骤 2: 加载并准备训练数据
我们需要一组已知的人脸图像作为训练数据。这里假设我们有一个名为`known_faces`的目录,其中包含了一些预先标记好的人脸图片。
def load_known_faces(directory):
known_face_encodings = []
known_face_names = []
for filename in os.listdir(directory):
image = face_recognition.load_image_file(os.path.join(directory, filename))
encoding = face_recognition.face_encodings(image)[0]
known_face_encodings.append(encoding)
known_face_names.append(filename.split(".")[0])
return known_face_encodings, known_face_names
known_face_encodings, known_face_names = load_known_faces("known_faces")
步骤 3: 实时摄像头捕获与识别
接下来,我们编写一个函数来从摄像头获取实时视频流,并对每一帧进行人脸识别。
def recognize_faces(frame):
# 调整图像大小以提高识别速度
small_frame = cv2.resize(frame, (0, 0), fx=0.25, fy=0.25)
# 将BGR颜色空间转换为RGB
rgb_small_frame = small_frame[:, :, ::-1]
# 在图像中查找所有人脸位置及其编码
face_locations = face_recognition.face_locations(rgb_small_frame)
face_encodings = face_recognition.face_encodings(rgb_small_frame, face_locations)
face_names = []
for face_encoding in face_encodings:
matches = face_recognition.compare_faces(known_face_encodings, face_encoding)
name = "Unknown"
# 如果找到匹配,则使用第一个
if True in matches:
matched_indices = [i for i, b in enumerate(matches) if b]
counts = {}
for index in matched_indices:
name = known_face_names[index]
counts[name] += 1
name = max(counts, key=counts.get)
face_names.append(name)
return face_locations, face_names
# 打开摄像头
video_capture = cv2.VideoCapture(0)
while True:
ret, frame = video_capture.read()
face_locations, face_names = recognize_faces(frame)
for (top, right, bottom, left), name in zip(face_locations, face_names):
# 因为调整了图像大小,所以需要放大坐标
top *= 4
right *= 4
bottom *= 4
left *= 4
# 画出框
cv2.rectangle(frame, (left, top), (right, bottom), (0, 0, 255), 2)
# 画出标签
cv2.rectangle(frame, (left, bottom - 35), (right, bottom), (0, 0, 255), cv2.FILLED)
font = cv2.FONT_HERSHEY_DUPLEX
cv2.putText(frame, name, (left + 6, bottom - 6), font, 1.0, (255, 255, 255), 1)
# 显示结果图像
cv2.imshow('Video', frame)
# 按q键退出
if cv2.waitKey(1) & 0xFF == ord('q'):
break
# 释放摄像头资源
video_capture.release()
cv2.destroyAllWindows()
总结通过上述步骤,我们构建了一个简单但功能强大的人脸识别系统。该系统能够实时地从摄像头捕获视频,并识别出已知的人脸。此程序可用于多种应用场景,如安全监控、身份验证等。