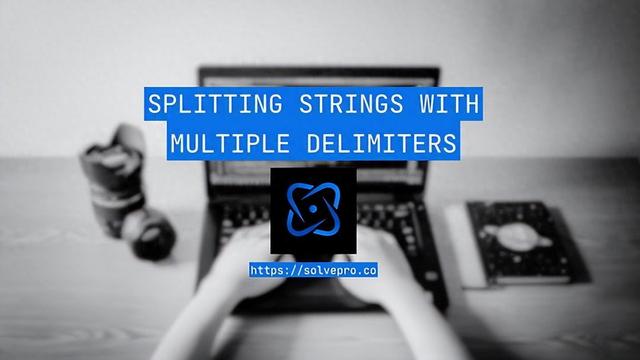
在 Python 中处理文本数据时,您通常需要使用多个分隔符将字符串分开。无论您是解析日志文件、处理带有嵌套字段的 CSV 数据,还是清理用户输入,了解如何有效地拆分字符串都是必不可少的。让我们探索您可以立即开始使用的实用解决方案。
将 str.split() 与多个步骤一起使用最直接的方法从 Python 的内置字符串拆分开始。虽然不是最优雅的解决方案,但它非常适合快速任务且易于理解:
# Split a string containing both commas and semicolonstext = "apple,banana;orange,grape;pear"# Split in two stepsstep1 = text.split(';') # First split by semicolonsresult = []for item in step1: result.extend(item.split(',')) # Then split by commasprint(result) # Output: ['apple', 'banana', 'orange', 'grape', 'pear']可以把这想象成剪一张纸:首先你沿着所有的水平线剪,然后你拿每条条带并沿着垂直线剪。这很简单,但如果你有许多不同的分隔符,就会变得乏味。
将 re.split() 用于多个分隔符're.split()' 函数就像一把聪明的剪刀,可以一次剪断多个图案。它比 str.split() 更复杂,可以轻松处理复杂的模式:
import re# Split by multiple delimiters using regular expressionstext = "apple,banana;orange|grape.pear"# The pattern [,;|.] means "cut wherever you see any of these characters"result = re.split('[,;|.]', text)print(result) # Output: ['apple', 'banana', 'orange', 'grape', 'pear']# When your delimiters include special characters, escape them to avoid errorstext_with_special = "apple$banana#orange@grape"result = re.split('[$#@]', text_with_special) # Each character becomes a splitting pointprint(result) # Output: ['apple', 'banana', 'orange', 'grape']在处理多个分隔符时,此方法要简洁得多。它不是对文本进行多次遍历,而是一次性处理所有内容。这就像拥有一个文档扫描仪,可以同时识别和拆分多种类型的标记。
处理具有多个分隔符的复杂数据现实世界的数据通常是杂乱的,并且是分层的。下面是一个实际示例,演示如何处理不同部分使用不同分隔符的日志文件:
import redef parse_log_line(line): """ Parse a log line with this format: timestamp|level|key1=value1;key2=value2,key3=value3 The structure breaks down like this: - Main sections are separated by pipes (|) - Data section contains key-value pairs - Key-value pairs are separated by semicolons (;) or commas (,) - Each pair uses equals (=) between key and value """ # Split the main sections first main_parts = re.split(r'\|', line, maxsplit=2) if len(main_parts) != 3: return None timestamp, level, data = main_parts # Process the data section into a dictionary data_parts = {} for item in re.split(';|,', data): # Split by either semicolon or comma if '=' in item: key, value = item.split('=', 1) # Split on first equals sign only data_parts[key.strip()] = value.strip() return { 'timestamp': timestamp.strip(), 'level': level.strip(), 'data': data_parts }# Example usagelog_line = "2024-01-28 15:30:45|ERROR|Module=Auth;User=john.doe,Status=failed"result = parse_log_line(log_line)print(result)# Output:# {# 'timestamp': '2024-01-28 15:30:45',# 'level': 'ERROR',# 'data': {# 'Module': 'Auth',# 'User': 'john.doe',# 'Status': 'failed'# }# }此示例说明如何像洋葱一样剥离数据 — 一次删除一层结构。我们首先拆分主要部分,然后使用其自己的一组分隔符处理 detailed data 部分。
在结果中保留分隔符有时,您不仅需要了解各个部分,还需要了解它们的区别。当您需要稍后重新构造字符串或自行处理分隔符时,这非常有用:
import redef split_keep_delimiters(text, delimiters): """ Split the text but preserve the characters that did the splitting. Like keeping track of where you made your cuts in a piece of paper. Args: text: The string to split delimiters: List of characters that should split the string """ # Create a pattern that captures (keeps) the delimiters # The parentheses in the pattern tell regex to include the matches in the result pattern = f'([{"".join(map(re.escape, delimiters))}])' # Split while keeping the delimiters in the result parts = re.split(pattern, text) # Remove empty strings that might occur between delimiters return [part for part in parts if part]# Example usagetext = "apple,banana;orange|grape"delimiters = [',', ';', '|']result = split_keep_delimiters(text, delimiters)print(result) # Output: ['apple', ',', 'banana', ';', 'orange', '|', 'grape']# Now you can put it back together differentlynew_text = ':'.join(result)print(new_text) # Output: apple:,:banana:;:orange:|:grape使用混合分隔符处理类似 CSV 的数据以下是如何处理对不同级别信息使用不同分隔符的数据,例如某些单元格包含列表的电子表格:
import redef parse_mixed_csv(text): """ Parse text that's structured like a CSV but with subcategories: - Rows are separated by newlines - Main fields are separated by commas - Some fields contain sublists separated by semicolons Example input: name,skills;level,location John Doe,Python;expert;SQL;intermediate,New York """ records = [] # Split into lines first (handling one row at a time) for line in text.strip().split('\n'): # Split fields by comma, but be smart about semicolons # The regex pattern looks for commas that have an even number of semicolons ahead fields = re.split(r',(?=[^;]*(?:;|$))', line) # Process each field processed_fields = [] for field in fields: # If the field contains semicolons, it's a sublist if ';' in field: subfields = field.split(';') processed_fields.append(subfields) else: processed_fields.append(field) records.append(processed_fields) return records# Example usagedata = """name,skills;level,locationJohn Doe,Python;expert;SQL;intermediate,New YorkJane Smith,Java;advanced;Python;beginner,London"""result = parse_mixed_csv(data)# Print in a readable formatfor record in result: print(record)# Output:# ['name', ['skills', 'level'], 'location']# ['John Doe', ['Python', 'expert', 'SQL', 'intermediate'], 'New York']# ['Jane Smith', ['Java', 'advanced', 'Python', 'beginner'], 'London']处理特殊情况和空字段真实数据是混乱的。以下是处理您在野外遇到的边缘情况的方法:
import redef smart_split(text, delimiters, keep_empty=False): """ A robust splitting function that handles common edge cases: - Multiple delimiters in a row (like "a,,b") - Extra whitespace around fields - Empty input strings - Preserving or removing empty fields Args: text: The string to split delimiters: List of characters to split on keep_empty: Whether to keep empty fields in the result """ # Build the regex pattern, escaping special characters pattern = '|'.join(map(re.escape, delimiters)) # Split the text parts = re.split(pattern, text) # Handle empty fields based on the keep_empty parameter if keep_empty: return parts return [part for part in parts if part.strip()]# Let's see how it handles tricky casesexamples = [ "apple,,banana;;orange||grape", # Multiple delimiters together " apple , banana ; orange ", # Messy whitespace ",,,", # String of just delimiters "" # Empty string]for example in examples: # Try both with and without empty fields with_empty = smart_split(example, [',', ';', '|'], keep_empty=True) print(f"With empty fields: {with_empty}") without_empty = smart_split(example, [',', ';', '|'], keep_empty=False) print(f"Without empty fields: {without_empty}") print()# Example output:# With empty fields: ['apple', '', 'banana', '', 'orange', '', 'grape']# Without empty fields: ['apple', 'banana', 'orange', 'grape']## With empty fields: [' apple ', ' banana ', ' orange ']# Without empty fields: ['apple', 'banana', 'orange']## With empty fields: ['', '', '', '']# Without empty fields: []## With empty fields: ['']# Without empty fields: []这些方法中的每一种都有其位置:- 使用 'str.split()' 进行快速、简单的拆分,其中可读性最重要- 当你需要一次在多个分隔符上进行拆分时,请使用 're.split()'- 当您需要对空字段、空格或嵌套结构进行特殊处理时,请使用自定义函数
选择与数据复杂性和代码需求相匹配的方法。最简单的有效解决方案通常是最好的选择。