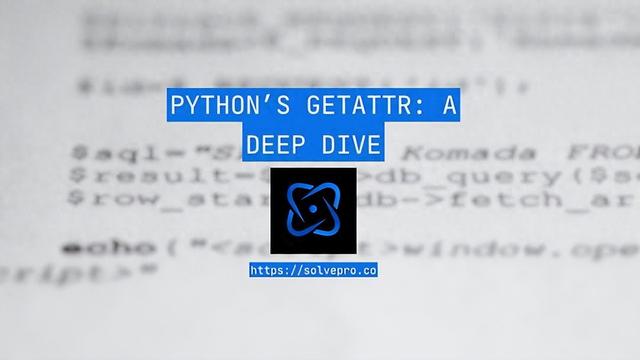
Python 的 'getattr()' 函数可能不会成为头条新闻,但它是一旦你了解如何使用它就变得不可或缺的功能之一。此内置函数允许您在运行时动态访问对象属性,从而为更灵活和可维护的代码提供了可能性。
getattr() 实际上是做什么的?在其核心,'getattr()' 使用字符串名称从对象中检索属性。以下是基本语法:
value = getattr(object, attribute_name, default_value)将其视为点表示法的功能等效项:
# These two lines do the same thingx = my_object.some_attributex = getattr(my_object, "some_attribute")但是,当点表示法更短时,为什么还要使用 'getattr()'呢?让我们深入研究一些 'getattr()' 大放异彩的真实示例。
动态属性访问的实际应用使用配置对象假设您正在构建一个游戏,其中角色统计数据是从用户配置加载的:
class Character: def __init__(self): self.strength = 10 self.dexterity = 8 self.intelligence = 12def apply_stat_boost(character, stat_name, boost): current_value = getattr(character, stat_name, 0) setattr(character, stat_name, current_value + boost)# Usagehero = Character()print(hero.strength) # Output: 10apply_stat_boost(hero, "strength", 5)print(hero.strength) # Output: 15在这里,'getattr()' 允许我们修改任何统计数据,而无需为每个统计数据编写单独的代码。我们甚至可以通过提供默认值来处理尚不存在的统计数据。
实现动态方法调用让我们看看 'getattr()' 如何帮助命令处理:
class TextEditor: def cut(self, text): return f"Cutting: {text}" def copy(self, text): return f"Copying: {text}" def paste(self, text): return f"Pasting: {text}" def execute_command(self, command, text): action = getattr(self, command, None) if action is None: return f"Unknown command: {command}" return action(text)# Usageeditor = TextEditor()print(editor.execute_command("copy", "Hello")) # Output: Copying: Helloprint(editor.execute_command("invalid", "Hello")) # Output: Unknown command: invalid错误处理和默认值'getattr()' 最有用的功能之一是它能够优雅地处理缺失的属性:
class User: def __init__(self, name): self.name = nameuser = User("Alice")# Without default value - raises AttributeErrortry: age = getattr(user, "age")except AttributeError: print("Age not found!")# With default value - returns 0 if age doesn't existage = getattr(user, "age", 0)print(age) # Output: 0实际应用动态数据处理使用具有变量字段的数据时:
class DataPoint: def __init__(self, **kwargs): for key, value in kwargs.items(): setattr(self, key, value) def get_field(self, field_name): return getattr(self, field_name, None)# Creating objects with different fieldspoint1 = DataPoint(temperature=20, humidity=45)point2 = DataPoint(pressure=1013, wind_speed=15)# Accessing fields uniformlyfields_to_check = ["temperature", "pressure", "humidity"]for point in [point1, point2]: values = [point.get_field(field) for field in fields_to_check] print(values) # Handles missing fields gracefully插件系统在构建插件系统时,'getattr()' 特别有用:
class Plugin: def __init__(self, name): self.name = name def initialize(self): print(f"Initializing {self.name}") def shutdown(self): print(f"Shutting down {self.name}")class PluginManager: def __init__(self): self.plugins = {} def add_plugin(self, plugin): self.plugins[plugin.name] = plugin def execute_action(self, plugin_name, action): plugin = self.plugins.get(plugin_name) if plugin: method = getattr(plugin, action, None) if method: method() else: print(f"Action {action} not supported by {plugin_name}") else: print(f"Plugin {plugin_name} not found")# Usagemanager = PluginManager()manager.add_plugin(Plugin("Database"))manager.add_plugin(Plugin("Cache"))manager.execute_action("Database", "initialize") # Output: Initializing Databasemanager.execute_action("Cache", "shutdown") # Output: Shutting down Cachemanager.execute_action("Database", "invalid") # Output: Action invalid not supported by Database常见问题和解决方案处理方法属性当对方法使用 'getattr()' 时,请记住你得到的是方法对象,而不是调用它的结果:
class Calculator: def add(self, a, b): return a + bcalc = Calculator()add_method = getattr(calc, "add")# This worksresult = add_method(5, 3) # Output: 8# This doesn't workresult = getattr(calc, "add")(5, 3) # More confusing to read类型 安全始终验证您获得的属性类型,尤其是在使用用户输入时:
class SafeAccess: def __init__(self): self.data = "sensitive info" self._private = "hidden" def get_attribute(self, name): attr = getattr(self, name, None) # Ensure we're not exposing private attributes if name.startswith('_'): return None # Ensure we're not exposing callable attributes if callable(attr): return None return attrobj = SafeAccess()print(obj.get_attribute("data")) # Output: sensitive infoprint(obj.get_attribute("_private")) # Output: None何时不使用 getattr()虽然 'getattr()' 很有用,但它并不总是最好的选择:
1. 如果在编码时知道属性名称,请使用常规点表示法2. 当您需要在紧密循环中访问许多属性时(点表示法更快)3. 当类型检查和代码完成至关重要时(IDE 无法通过动态访问推断类型)
超越基本用法'getattr()' 在与其他 Python 功能结合使用时确实显示了它的价值:
class APIEndpoint: def __init__(self, base_url): self.base_url = base_url def __getattr__(self, name): # Create endpoint handlers dynamically def handler(*args, **kwargs): return f"Calling {name} on {self.base_url} with {args} and {kwargs}" return handlerapi = APIEndpoint("https://api.example.com")# These methods don't exist, but __getattr__ creates themprint(api.get_user(123))print(api.create_post(title="Hello"))此模式对于创建 Fluent 接口或处理动态 API 端点特别有用。
请记住,虽然 'getattr()' 为您提供了灵活性,但这也意味着您失去了 Python 的一些静态分析优势。当您的代码的动态特性真正需要它时使用它,并很好地记录您的代码以帮助其他人理解您的意图。